leetCode-19(删除链表的倒数第N个结点)
给你一个链表,删除链表的倒数第 n
个结点,并且返回链表的头结点。
示例 1:
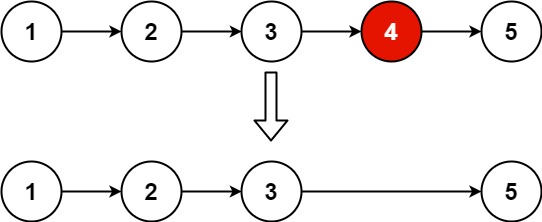
1 2
| 输入:head = [1,2,3,4,5], n = 2 输出:[1,2,3,5]
|
示例 2:
示例 3:
解法一,自己瞎写
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
|
class Solution { public ListNode removeNthFromEnd(ListNode head, int n) { ListNode node = head; int count = 0; while (node!=null){ node = node.next; count++; } if (count==0) return head; if (count==1) return null; node = head; if (count-n==0) { head = head.next; return head; } for (int i = 1;i<count-n;i++){ node = node.next; } node.next = node.next.next; node = head; return node; } }
|
解法二:栈
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
class Solution { public ListNode removeNthFromEnd(ListNode head, int n) { ListNode dummy = new ListNode(0, head); Stack<ListNode> stack = new Stack<>(); ListNode node = dummy; while (node!=null){ stack.push(node); node = node.next; } for (int i = 1;i<=n;i++){ stack.pop(); } ListNode pre = stack.peek(); pre.next = pre.next.next; return dummy.next; } }
|
不用辅助节点:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
class Solution { public ListNode removeNthFromEnd(ListNode head, int n) { Stack<ListNode> stack = new Stack<>(); ListNode node = head; while (node!=null){ stack.push(node); node = node.next; } for (int i = 1;i<=n;i++){ stack.pop(); } if (stack.size()==0) return head.next; node = stack.pop(); node.next = node.next.next; return head; } }
|
解法三:双指针
- 定义两个指针,一个first,一个second。
- first指针先走n步
- 再将first和second两个指针,同时后移,直到first为null。
- 则second指向的是倒是n+1个节点。
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
class Solution { public ListNode removeNthFromEnd(ListNode head, int n) { ListNode dummy = new ListNode(0,head); ListNode first = head; ListNode second = dummy; for (int i = 1;i<=n;i++){ first = first.next; } while (first!=null){ first = first.next; second = second.next; } second.next = second.next.next; ListNode node = dummy.next; return node; } }
|
不用辅助节点时:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
class Solution { public ListNode removeNthFromEnd(ListNode head, int n) { ListNode first = head; ListNode second = head; for (int i = 1;i<=n;i++){ first = first.next; } if (first==null) return head.next; while (first.next!=null){ first = first.next; second = second.next; } second.next = second.next.next; return head; } }
|