leetCode–226(翻转二叉树)
给你一棵二叉树的根节点 root
,翻转这棵二叉树,并返回其根节点。
示例 1:
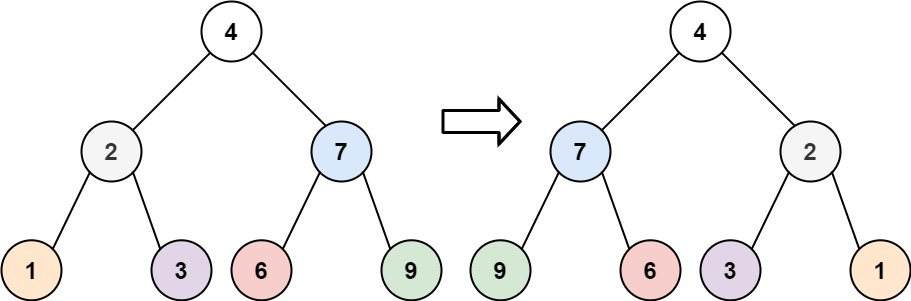
1 2
| 输入:root = [4,2,7,1,3,6,9] 输出:[4,7,2,9,6,3,1]
|
示例 2:
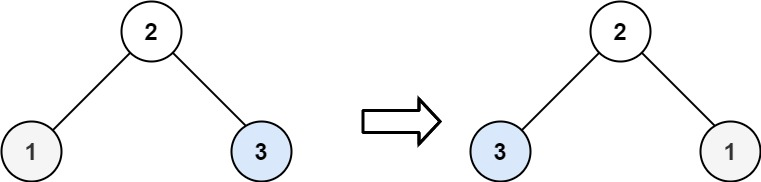
示例 3:
解法一:递归
遍历每个节点,转换每个节点的左右子树。这个想法要是想到了,代码会很简单,但没想到!
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
class Solution { public TreeNode invertTree(TreeNode root) { if (root==null) return null; TreeNode t = root.left; root.left = root.right; root.right = t; if (root.left!=null) invertTree(root.left); if (root.right!=null) invertTree(root.right); return root; } }
|
解法二:迭代
额外的数据结构–队列,来存放临时遍历到的元素。
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
|
class Solution { public TreeNode invertTree(TreeNode root) { if (root==null) return null; Stack<TreeNode> stack = new Stack<>(); stack.push(root); while (!stack.isEmpty()){ TreeNode a = stack.pop(); TreeNode t = a.left; a.left = a.right; a.right = t; if (a.left!=null) stack.push(a.left); if (a.right!=null) stack.push(a.right); } return root; } }
|