leetCode–160(相交链表)
给你两个单链表的头节点 headA 和 headB ,请你找出并返回两个单链表相交的起始节点。如果两个链表不存在相交节点,返回 null 。
图示两个链表在节点 c1 开始相交:
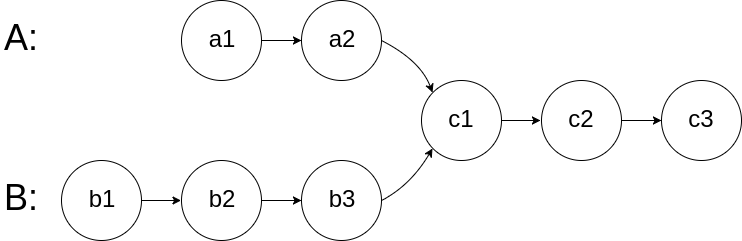
题目数据 保证 整个链式结构中不存在环。
注意,函数返回结果后,链表必须 保持其原始结构 。
自定义评测:
评测系统 的输入如下(你设计的程序 不适用 此输入):
- intersectVal - 相交的起始节点的值。如果不存在相交节点,这一值为 0
- listA - 第一个链表
- listB - 第二个链表
- skipA - 在 listA 中(从头节点开始)跳到交叉节点的节点数
- skipB - 在 listB 中(从头节点开始)跳到交叉节点的节点数
评测系统将根据这些输入创建链式数据结构,并将两个头节点 headA 和 headB 传递给你的程序。如果程序能够正确返回相交节点,那么你的解决方案将被 视作正确答案 。
示例 1:
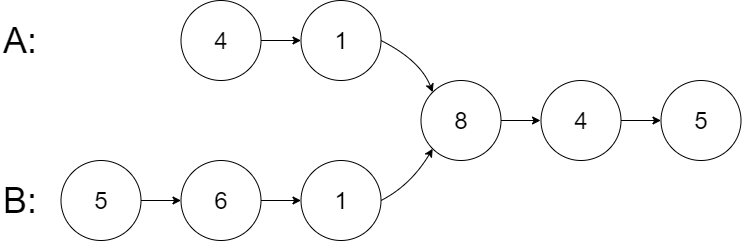
1 2 3
| 输入:intersectVal = 8, listA = [4,1,8,4,5], listB = [5,6,1,8,4,5], skipA = 2, skipB = 3 输出:Intersected at '8' 解释:相交节点的值为 8 (注意,如果两个链表相交则不能为 0)。从各自的表头开始算起,链表 A 为 [4,1,8,4,5],链表 B 为 [5,6,1,8,4,5]。在 A 中,相交节点前有 2 个节点;在 B 中,相交节点前有 3 个节点。
|
示例 2:
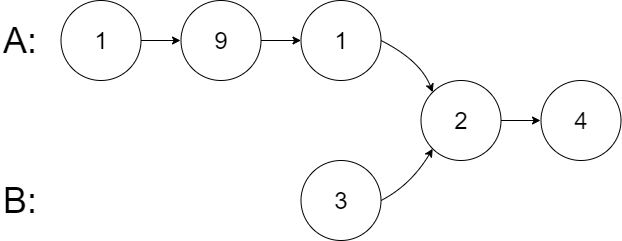
1 2 3
| 输入:intersectVal = 2, listA = [1,9,1,2,4], listB = [3,2,4], skipA = 3, skipB = 1 输出:Intersected at '2' 解释:相交节点的值为 2 (注意,如果两个链表相交则不能为 0)。从各自的表头开始算起,链表 A 为 [1,9,1,2,4],链表 B 为 [3,2,4]。在 A 中,相交节点前有 3 个节点;在 B 中,相交节点前有 1 个节点。
|
示例 3:
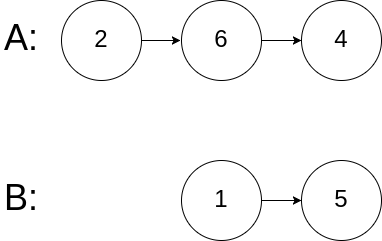
1 2 3
| 输入:intersectVal = 0, listA = [2,6,4], listB = [1,5], skipA = 3, skipB = 2 输出:null 解释:从各自的表头开始算起,链表 A 为 [2,6,4],链表 B 为 [1,5]。由于这两个链表不相交,所以 intersectVal 必须为 0,而 skipA 和 skipB 可以是任意值。这两个链表不相交,因此返回 null 。
|
解法一:哈希表
遍历链表A,将链表A中的元素存储到list集合中,再遍历链表B,如果遇到相等的节点,说明A和B相交了,如果没有相等的则返回null。
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
public class Solution { public ListNode getIntersectionNode(ListNode headA, ListNode headB) { List<ListNode> list = new ArrayList<>(); ListNode a = headA; while (a!=null){ list.add(a); a = a.next; } a = headB; while (a!=null){ if (list.contains(a)){ return a; } a = a.next; } return null; } }
|
解法二:双指针
这个方法太巧妙了 ,,但看了好半天才看懂,太不好想了~~什么时候能一下就想出来这么好的解法呢。
先定义两个指针a 和 b。
a指向链表A的头结点,b指向链表B的头结点。
如果a为空说明链表A遍历完成,再让 a 指向 链表B的头结点,继续遍历。
如果 b 为空说明链表B遍历完成,再让b 指向链表A的头结点,继续遍历。
也就是说:
设链表A的节点数为 A ,设链表B的节点数为 B ,相交重合的节点数为 C。
- 指针
A
先遍历完链表 headA
,再开始遍历链表 headB
,当走到 node
时,共走步数为:a+(b−c)
- 指针
B
先遍历完链表 headB
,再开始遍历链表 headA
,当走到 node
时,共走步数为:b+(a−c)
此时 指针a和指针b重合,
如果两个链表相交:同时指向 node;
如果两个链表不相交:a和b同时为null;
用图来表示:
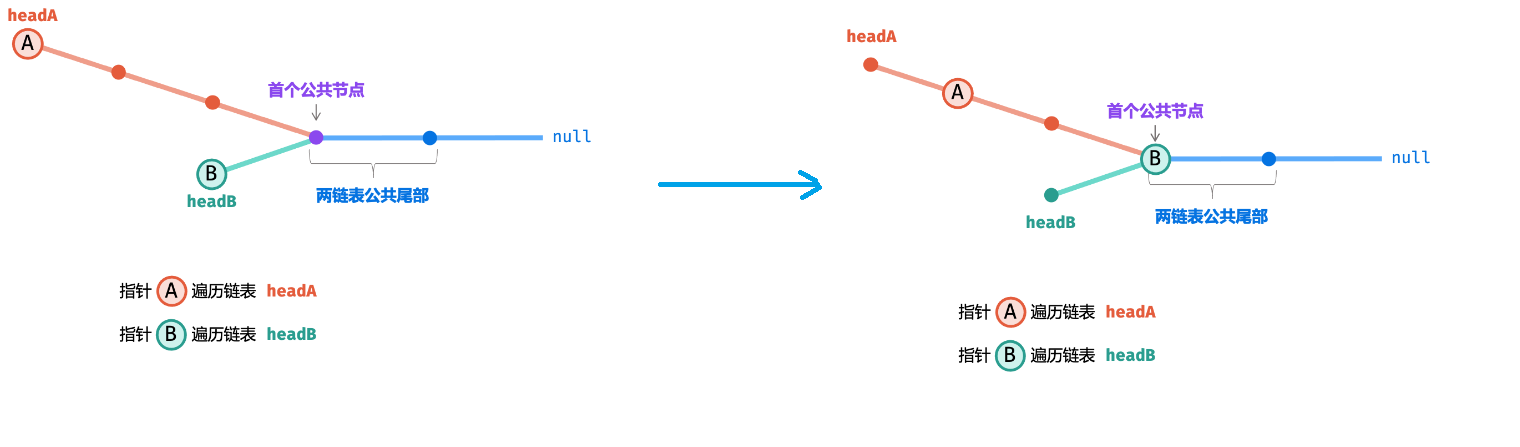
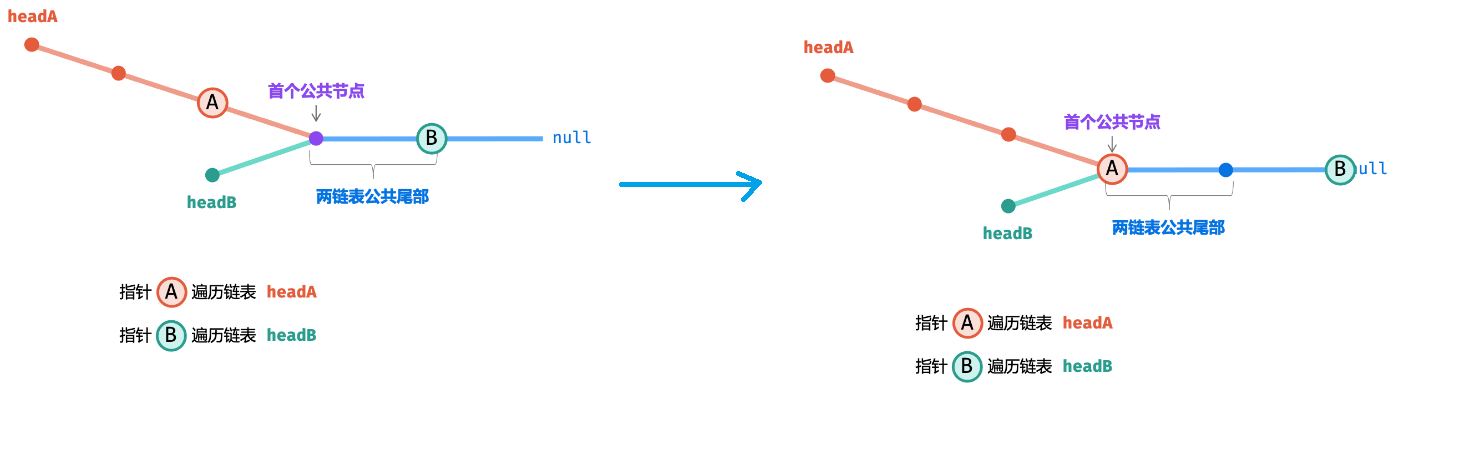
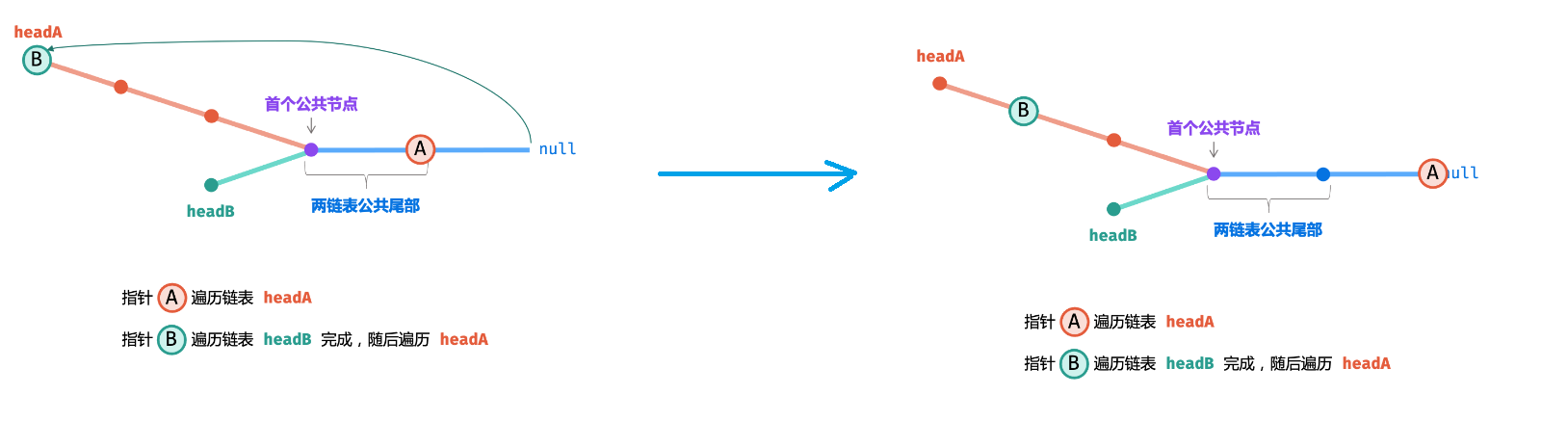
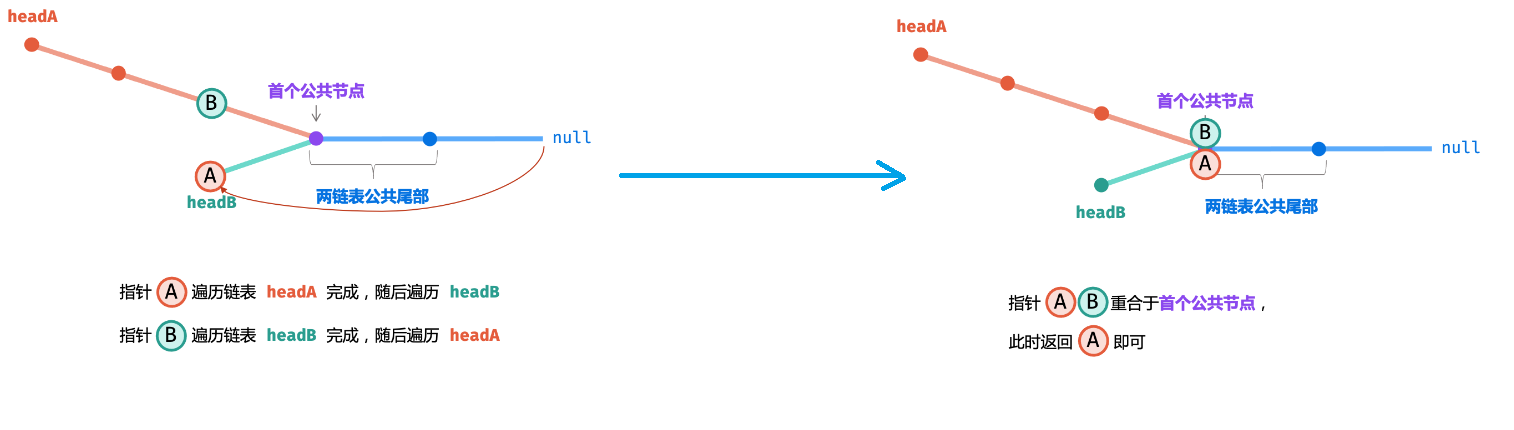
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
|
public class Solution { public ListNode getIntersectionNode(ListNode headA, ListNode headB) { if (headA==null ||headB==null) return null; ListNode a = headA; ListNode b = headB; while (a!=b){ a = a==null?headB:a.next; b = b==null?headA:b.next; } return a; } }
|