leetCode–206(反转链表)
给你单链表的头节点 head ,请你反转链表,并返回反转后的链表。
示例 1:
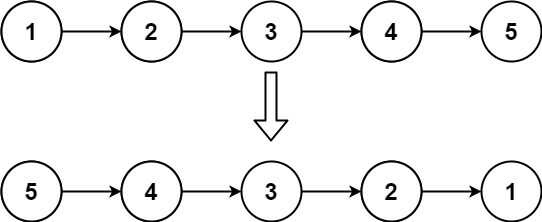
1 2
| 输入:head = [1,2,3,4,5] 输出:[5,4,3,2,1]
|
示例 2:
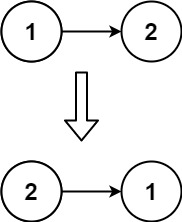
示例 3:
解法一:迭代
定于两个指针,第一个指针pre指向null,第二个cur指针指向head。
不断的遍历cur,终止条件是:cur为空。
每次遍历,将cur指向pre。之后pre等于cur。cur向后移动一位。以此类推。
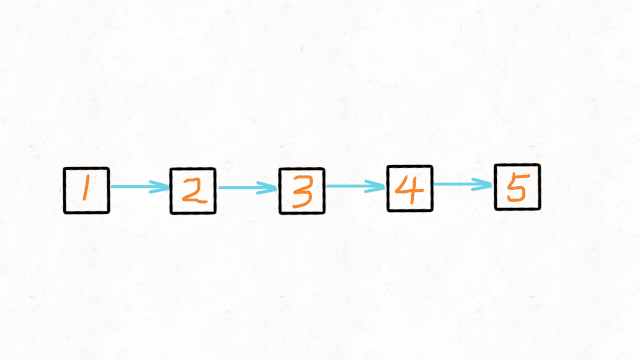
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
|
class Solution { public ListNode reverseList(ListNode head) { ListNode cur = head; ListNode pre = null; ListNode next = null; while(cur!=null){ next = cur.next; cur.next = pre; pre = cur; cur = next; } return pre; } }
|
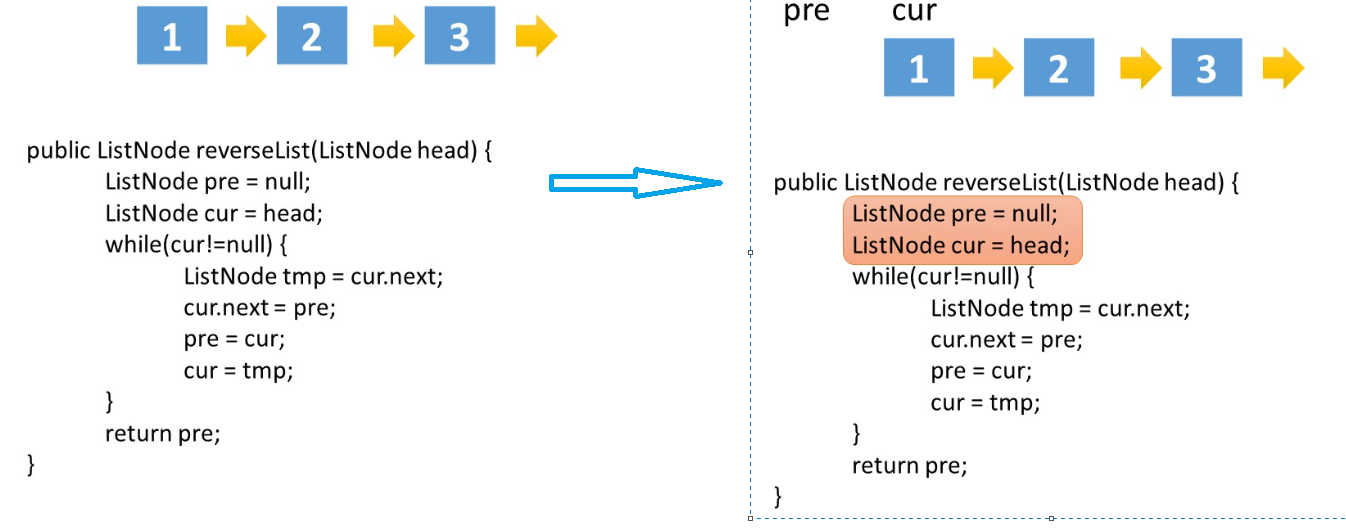
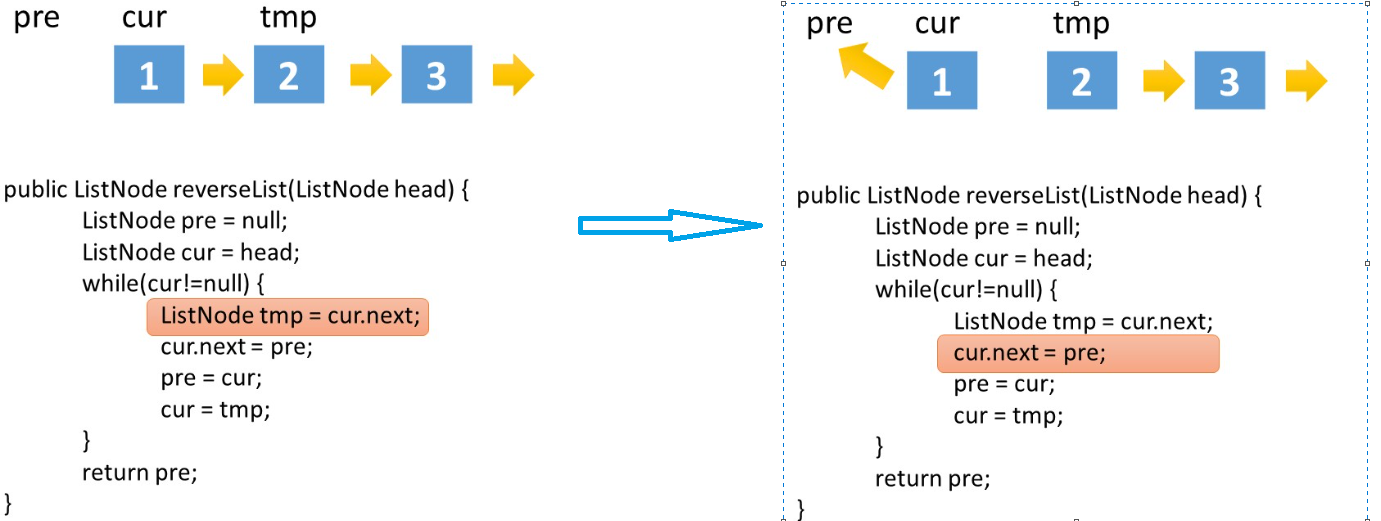
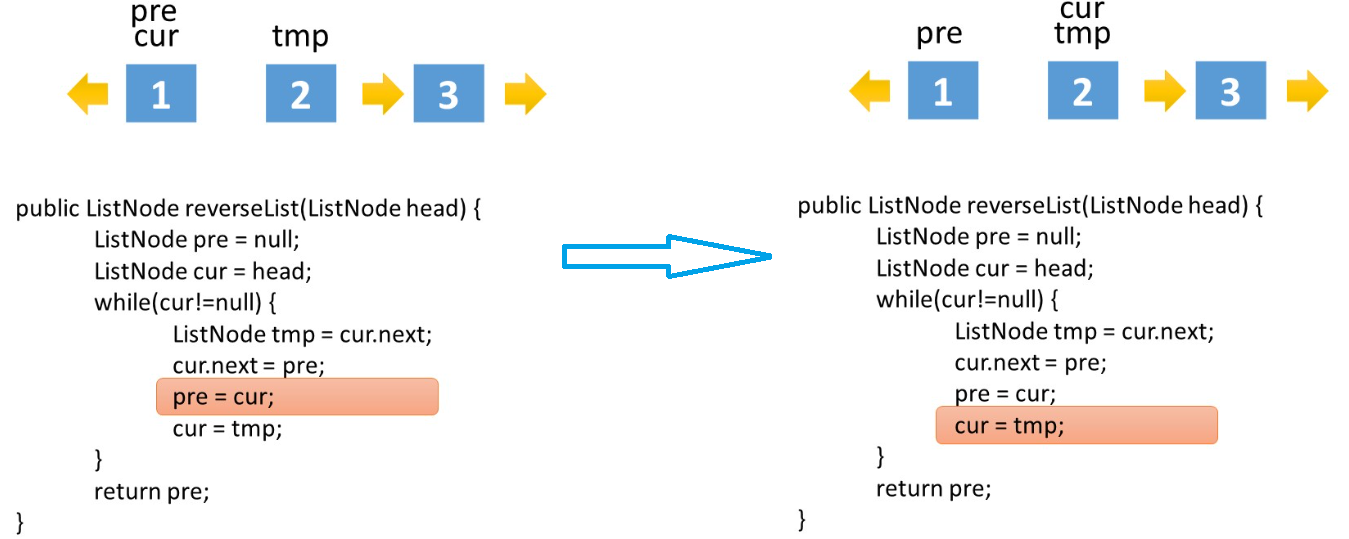
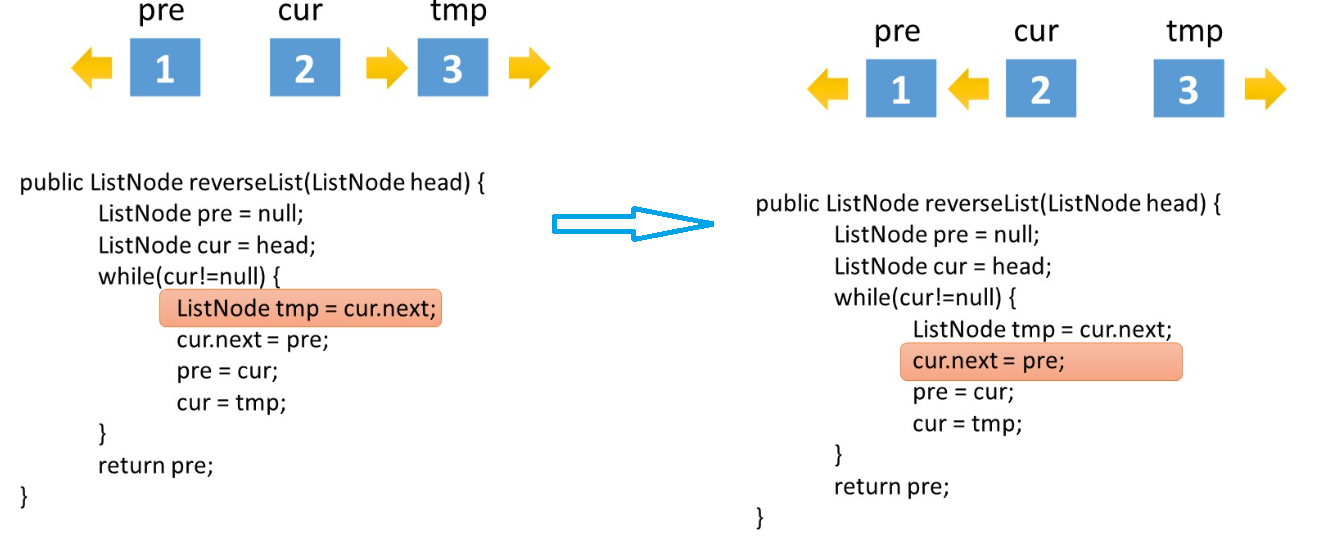
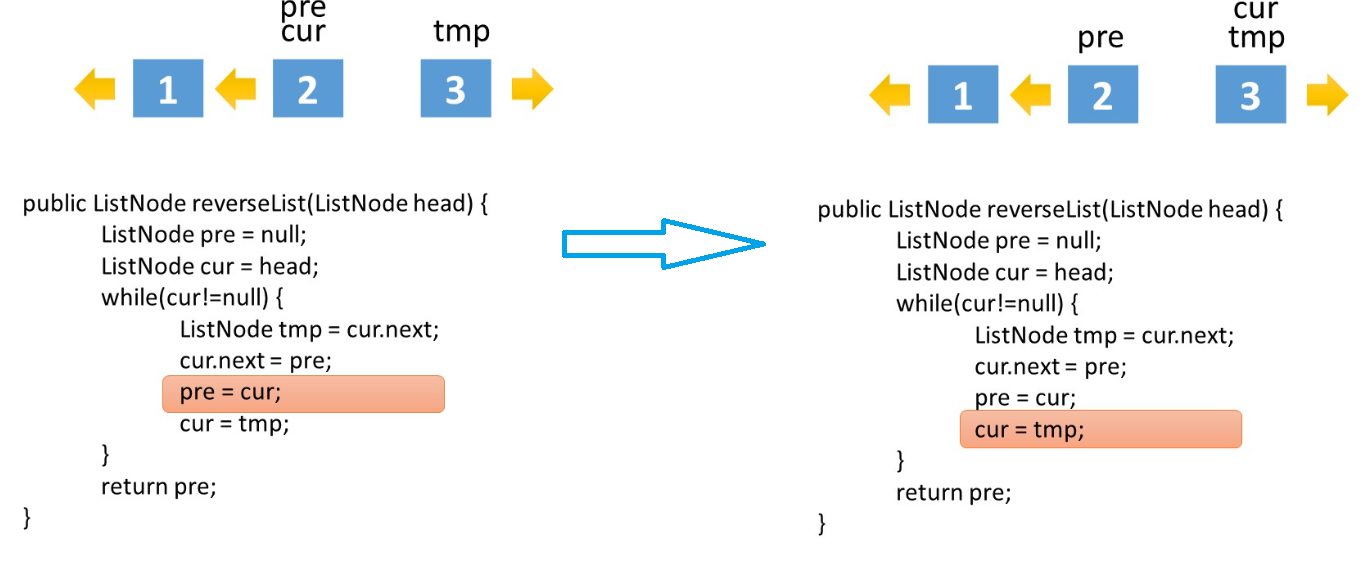
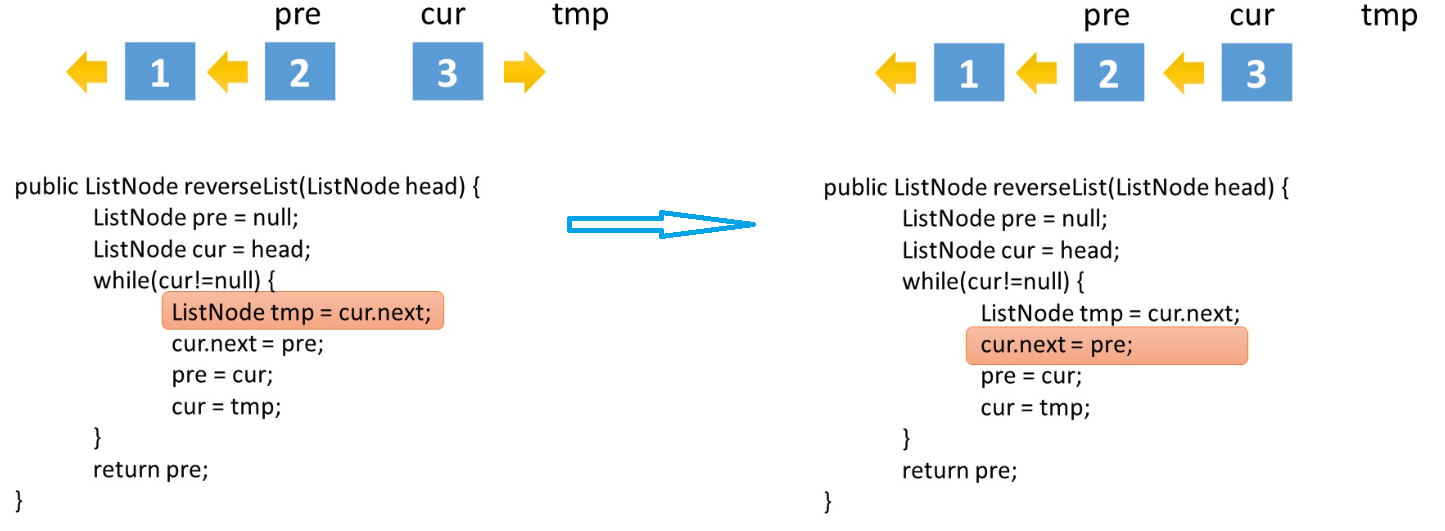
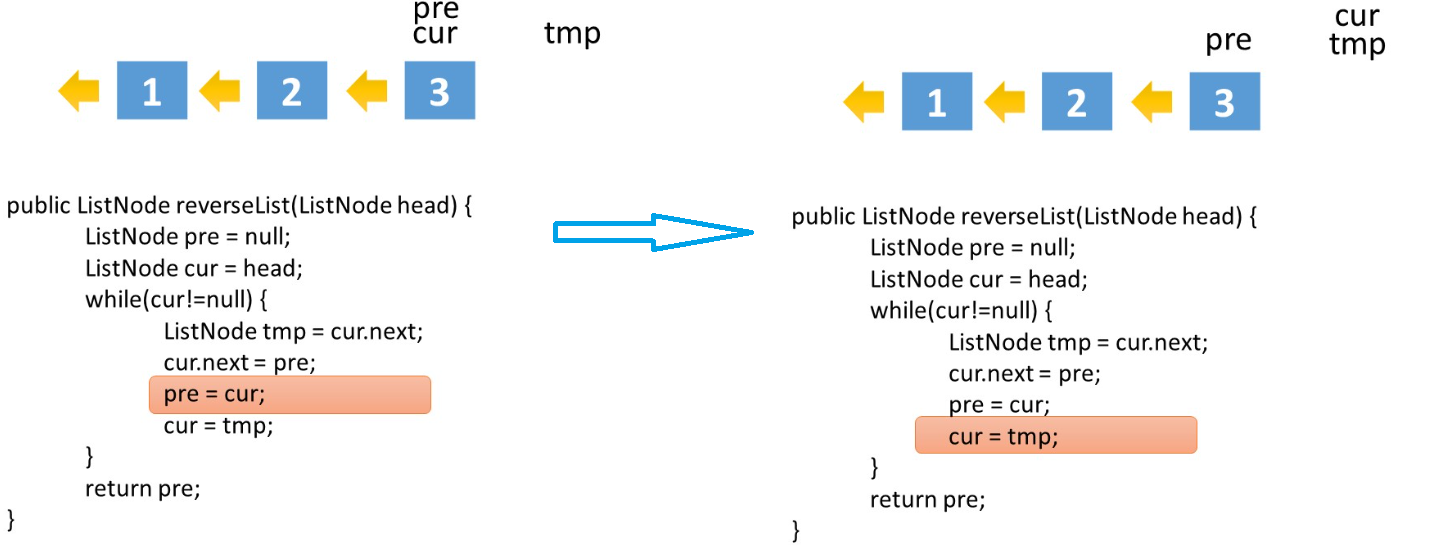
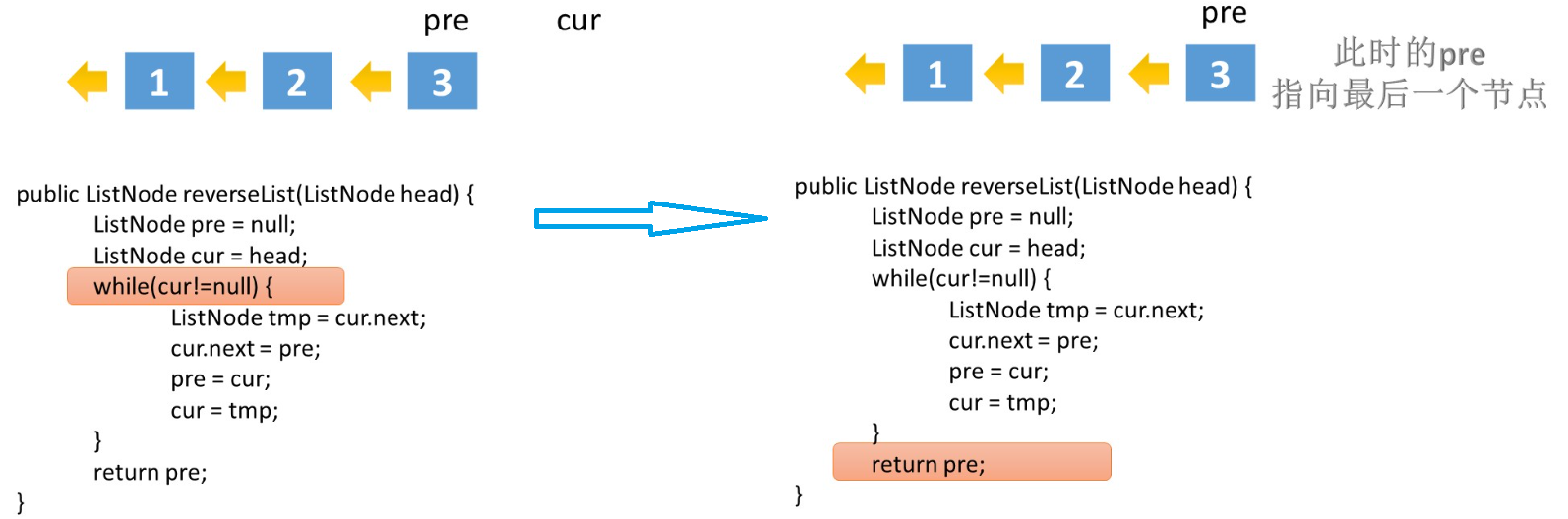
解法二:递归
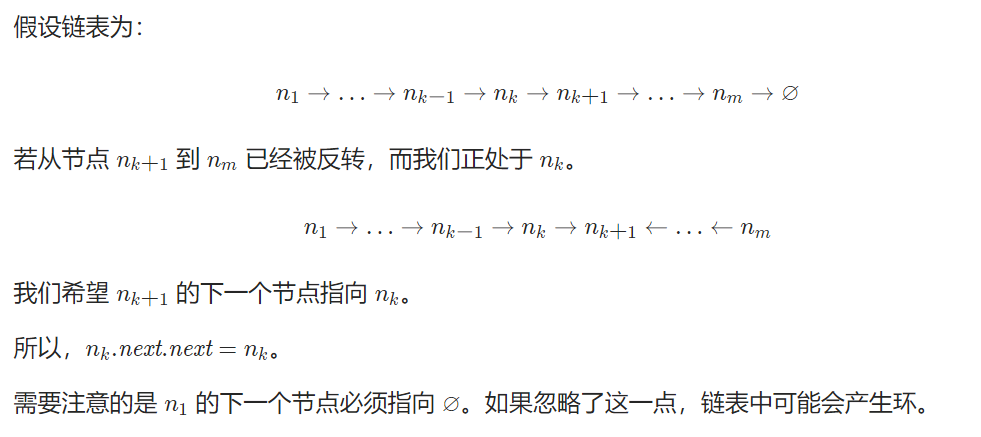
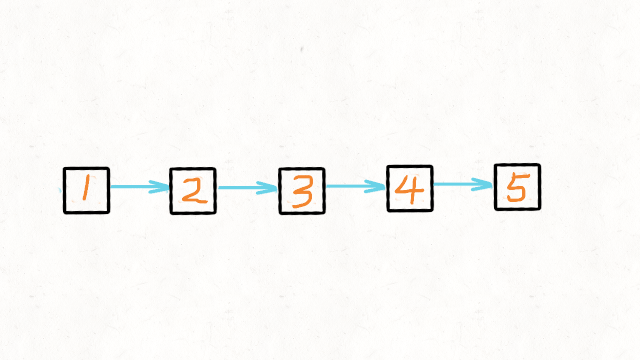
递归的两个条件:
- 终止条件是当前节点或者下一个节点==null
- 在函数内部,改变节点的指向,也就是 head 的下一个节点指向 head 递归函数那句
递归函数中每次返回的 cur 其实只最后一个节点,在递归函数内部,改变的是当前节点的指向。
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
class Solution { public ListNode reverseList(ListNode head) { if (head==null || head.next==null) return head; ListNode newNode = reverseList(head.next); head.next.next = head; head.next = null; return newNode; } }
|