leetCode–101(简单)
给你一个二叉树的根节点 root , 检查它是否轴对称。
示例 1:
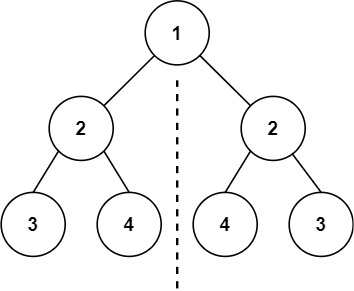
1 2
| 输入:root = [1,2,2,3,4,4,3] 输出:true
|
示例 2:
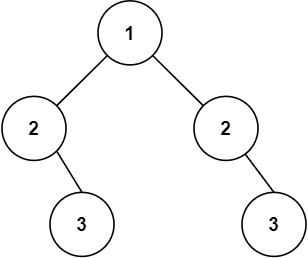
1 2
| 输入:root = [1,2,2,null,3,null,3] 输出:false
|
解法一:自己
哈哈哈哈,笑死了 我这算是什么解法~~
前序遍历,后序遍历,之后将后序遍历逆转,比较两个list是否相等。后来发现使用我这种方法,[1,2,2,null,3,null,3]这个用例过不去,因此将null值也加入了进去,瞎整~~过了。太菜了太菜了 不要看这个了 ,还是看大佬们的题解把
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
|
class Solution { TreeNode pre = new TreeNode(); TreeNode last = new TreeNode(); List<Integer> prelist = new ArrayList<>(); List<Integer> laselist = new ArrayList<>(); public boolean isSymmetric(TreeNode root) { if (root.left==null && root.right==null) return true; if (root.left==null || root.right==null) return false; pre = root; last = root; if (pre!=null){ preSort(pre); } if (last!=null){ lastSort(last); } Collections.reverse(laselist); return prelist.equals(laselist); } public void preSort(TreeNode root){ if (root!=null){ prelist.add(root.val); if (root.left!=null) preSort(root.left); else prelist.add(null); if (root.right!=null) preSort(root.right); else prelist.add(null); } } public void lastSort(TreeNode root){ if (root!=null){ if (root.left!=null) lastSort(root.left); else laselist.add(null); if (root.right!=null) lastSort(root.right); else laselist.add(null); laselist.add(root.val); } } }
|
解法二:递归
如果一个数是对称的,则左子树和右子树互为镜像。因此可以转化为,两个树在什么情况下互为镜像?
两个树互为镜像?
- 它们的两个根结点具有相同的值
- 每个树的右子树都与另一个树的左子树镜像对称
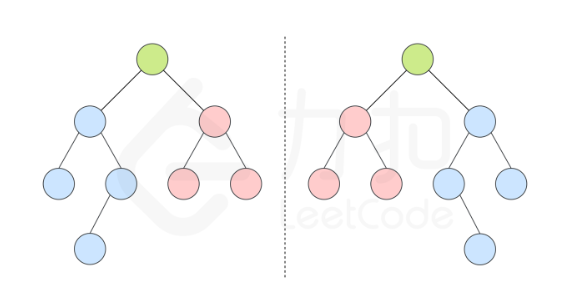
也就是将一个树变成两个树进行比较。
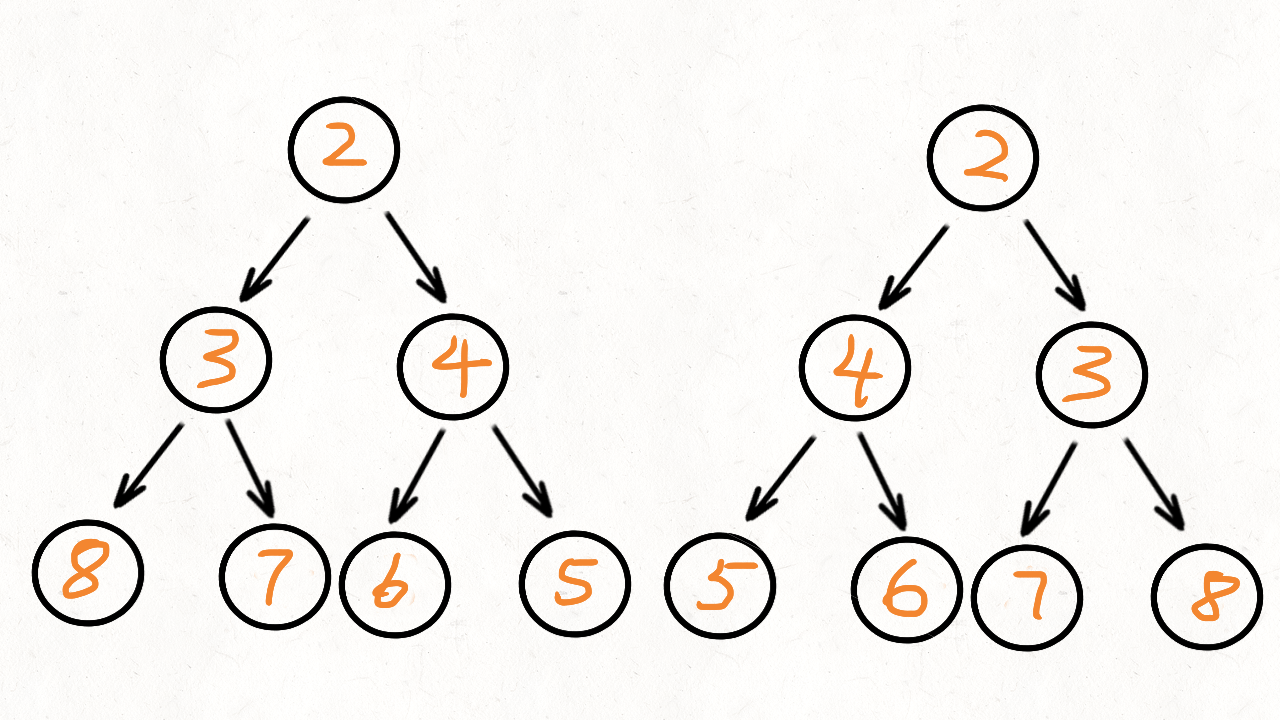
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
|
class Solution { public boolean isSymmetric(TreeNode root) { if(root==null) return true; return check(root, root); } public boolean check(TreeNode p,TreeNode q){ if (p==null && q==null) return true; if (p==null || q==null) return false; if (p.val==q.val) { return check(p.left,q.right) && check(p.right,q.left); }else return false; } }
|
解法三:迭代
将递归程序转换为迭代程序,最常用的方法就是引入一个队列。
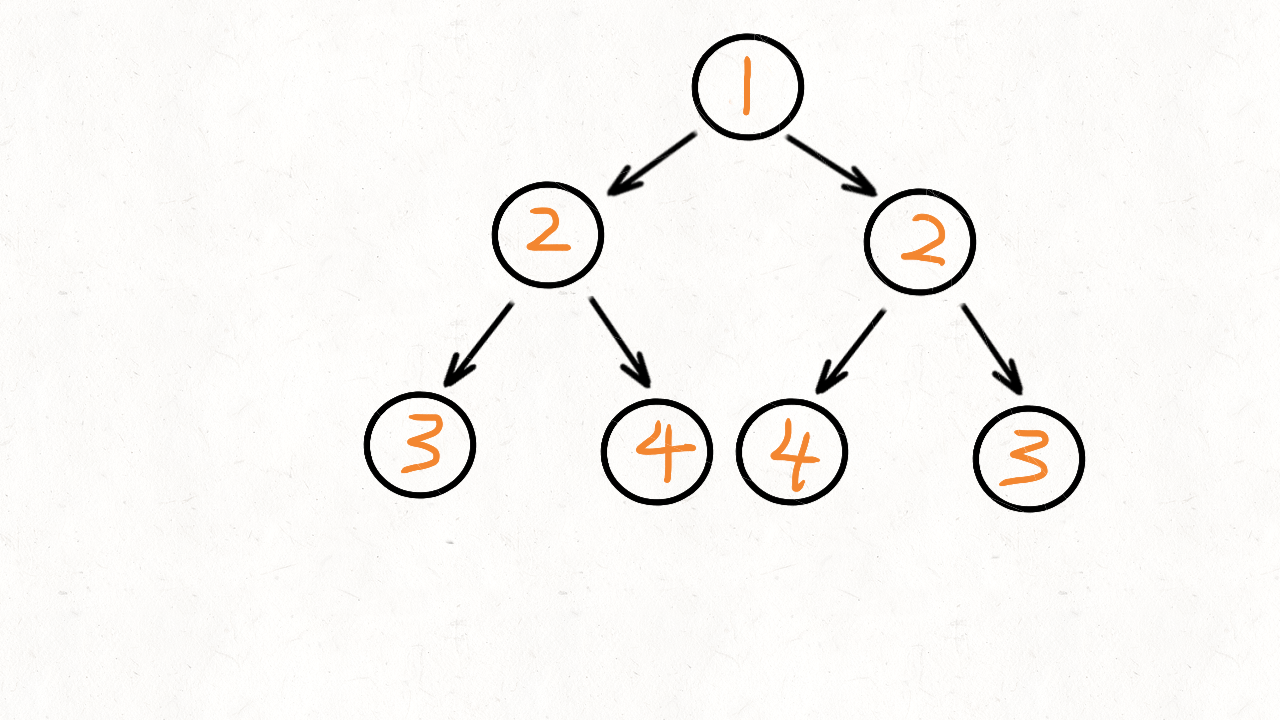
代码为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
|
class Solution { public boolean isSymmetric(TreeNode root) { if (root==null) return true; if (root.left==null && root.right==null) return true; if (root.left==null || root.right==null) return false; Deque<TreeNode> deque = new LinkedList<>(); deque.addLast(root.left); deque.addLast(root.right); while (!deque.isEmpty()){ TreeNode l = deque.removeFirst(); TreeNode r = deque.removeFirst(); if (l==null && r==null) continue; if (l==null || r==null) return false; if (l.val!=r.val) return false; deque.addLast(l.left); deque.addLast(r.right); deque.addLast(l.right); deque.addLast(r.left); } return deque.isEmpty(); } }
|